Concepts of Computer Science II
Records and Arrays
The purpose of this assignment is to introduce the concept of records.
The assignment is split into three parts. Each part can be done in
a single lab session. The total assignment is worth 15 points.
An array is able to store a great many numbered elements of the
same data type. A record is able to store several named elements
of different data types. See your text for a more complete explanation
of records. An example of a record type declaration would be:
The type ClassType is, of course an enumerated type which we discussed
earlier. Student is a record type. Name, Classification, Gender,
and GPA are components of the record and are treated much like the
components of classes. Following are two variable declarations involving
the record type Student.
var NewStudent: Student;
// declares a single object of type student.
ClassRoster: Array [1..50] of Student;
// declares an array of 50 students.
The following are some examples of the uses of the record types:
-
NewStudent.Name := NameEdit.text; // assigns a
value to the Name component of NewStudent.
-
Writeln(OutFile, NewStudent.GPA); // writes the GPA component
of NewStudent to the logical file OutFile.
-
NewStudent.Name := ClassRoster[15].Name; // assigns the name of the
15th student to NewStudent.
-
NewStudent is of type Student
-
NewStudent.Name is of type String
-
ClassRoster is an array of Student
-
ClassRoster[J] is of type Student
-
ClassRoster[J].Name is of type String.
-
for J := 1 to LastStudent do
StudentMemo.lines.add(ClassRoster[J].Name +
' ' + FloatToStr(ClassRoster[J].GPA);
This statement will write all of the names and GPAs of ClassRoster
(up to LastStudent) into the StudentMemo.
The WITH statement
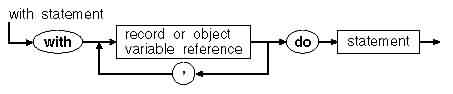
The with statement statement as a short hand notation when the same object
is referenced many times throughout a section of Pascal code. The
form of a with statement is indicated in the above example. The first
section of the following code is an example of a with statement.
The next section is the same code without a with statement.
Using a WITH statement
with OrderMemo do
begin
clear;
lines.add('Crawford''s Computer
System');
lines.add('
Complete System');
lines.add('Cost: $3,120.00');
end;
The same actions, programmed without a WITH statement
OrderMemo.clear;
OrderMemo.lines.add('Crawford''s
Computer System');
OrderMemo.lines.add('
Complete System');
OrderMemo.lines.add('Cost:
$3,120.00'); |
Note: The with statement must be used with care. Since components
of objects can have the same name as components of other objects the use
of the with statement can be confusing to someone who is trying to read
your code. There are those who say that the with statement should
never be used.
Part 1 -- Writing and reading a single record
-
Create a separate unit (not a form.)
-
In the interface part declare a record type. Use the text and the
example above as guides as how to declare a record, but chose your own
components. It should be anything you would like to save on a file.
It could deal with sports teams, your family, your friends, or anything
other topic.
-
Also in the interface part declare two procedures that would read and write
the record to an open text file. An example, using the record type
Student, would be
procedure WriteStudent(var F: TextFile; const
S: Student);
procedure ReadStudent(var F: TextFile; var S:
Student);
-
In the implementation part of the unit write the implementations of your
read and write procedures. An example, using the previous declarations,
would be:
procedure WriteStudent(var F: TextFile; const
S: Student);
begin
writeln(F, S.Name);
writeln(F, ClassTypeToStr(S.Classification));
if S.Gender then
writeln(F, 'M')
else
writeln(F, 'F');
writeln(F, S.GPA);
end; // WriteStudent
procedure ReadStudent(var F: TextFile; var S:
Student);
var TempString: String;
begin
readln(F, S.Name);
readln(F, TempString);
S.Classification := StrToClassType(TempString);
readln(F, TempString);
if TempString = 'M' then
S.Gender := true
else
S.Gender := false;
readln(F, S.GPA);
end; // ReadStudent
Two things you need to keep in mind. The first is that the order
the components are read and the order they are written must be the same.
The second is that the functions ClassTypeToStr and StrToClassType would
need to be written much like the string to Position functions were written
in an earlier lab.
-
In your main form have edit boxes for each component in your record.
Have two buttons (or menu items.) When the write button is clicked
copy the content of the edit boxes to a record variable then write the
record using your write procedure. When the read button is clicked
read the record using your read procedure then copy the components of the
record to the proper edit boxes. An example of the write procedure
would be:
procedure MainForm.WriteButtonClick(var Sender:
TObject);
var OutFile: TextFile;
NewStudent:
Student;
begin
if SaveDialog.execute then
begin
// open file
AssignFile(OutFile, SaveDialog.filename);
Rewrite(OutFile);
// transfer contents of edit boxes
to records
NewStudent.Name := NameEdit.text;
NewStudent.Classification := StrToClassType(ClassEdit.text);
NewStudent.Gender := (GenderEdit.text[1]
= 'M');
NewStudent.GPA := StrToFloat(GPAEdit.text);
// write the NewStudent record
WriteStudent(OutFile, NewStudent);
// close the file
CloseFile(OutFile);
end;// if SaveDialog
end; // WriteButtonClick
This part will be complete when you can write to a file then read from
the same file and retreive exactly the save values that were supposed to
be written.
Part 2 -- Writing and retreiving several records in a text file.
Modify part 1 so that instead of writing only one record, you will be writing
several records to a file.
-
Add a component FileName to the private part of the main form declaration.
Its type should be string.
-
Add a button or menu item captioned "New File".
-
In the OnClick event handler of the New File button, let the user chose
a new file name, set that file name to the FileName component declared
in item one. Also indicate in the caption of the main form the name
of the chosen file. Then open the file for output and close the file.
This will create an empty file on your disk, which will allow you to create
a new file with completely new records. Warning: The use of
this will delete any existing file with the chosen file name.
-
Add a button or menu item captioned "Old File". On its OnClick event
handler let the user chose a file name and assign it to the FileName.
Indicate in the caption of the main form the name of the chosen file.
You need not open and close the file.
-
Change the code on the write button or menu item and OnClick handler as
follows:
-
Change the caption to "New Record". You might also change its name.
-
Do not use a Save Dialog. Instead use the file name that is in the
FileName component of the main form.
-
Instead of Rewrite(filevariable) use Append(filevariable).
This will add a new record to the file instead of creating a new file.
-
Change the code on the read button or menu item to view the records on
a file in a memo box. The code might look something like this:
procedure MainForm.ReadButtonClick(var Sender:
TObject);
var
begin
if OpenDialog.execute then
begin
// open StudentFile for input
AssignFile(StudentFile, OpenDialog.filename);
Reset(StudentFile);
// read all records in StudentFile
and put them in Memo
while not eof(StudentFile) do
begin
ReadStudent(StudentFile,
CurrentStudent);
Memo.lines.add(CurrentStudent.Name
+ ' '
+ FloatToStr(CurrentStudent.GPA));
end; // while
// close StudentFile
CloseFile(StudentFile);
end; // ReadButtonClick
You will be done with part 2 when you can write several records to a
file then view them correctly in a memo box.
Part 3 -- Sorting arrays of records.
In this part you will sort the records you saved in part 2. You will
need two more buttons (or menu items). They will each sort by a different
field. It would be nice if one were sorted in ascending order while
the other sorted in descending order. The event handler of each button
will have three major parts:
-
Read the file into an array of records. You will have to open and
close the file, etc., but the primary read loop will be something like
the following:
Count := 0; // count the number of records
while not eof(YourFile) do
ReadYourRecord(YourFile, YourArray[Count];
YourFile will be the text file you have declared and opened for input.
YourArray will be the array of records you have declared. And ReadYourRecord
will be the read procedure you declared in your separate unit.
-
Sort the array of records. Use the insert sort. The only change
you will need to make from unit 11 is in the comparison of array types.
-
Write the contents of the sorted array back out to the file. You
will need to open the file for output (thereby deleting the old contents.)
The primary write process will be similar to:
for J := 1 to Count do
WriteYourRecord(YourFile, YourArray[J]);
Author: Albert
L. Crawford
Copyright notice